H2 데이터베이스 설치 순수 Jdbc 스프링 통합 테스트 스프링 JdbcTemplate JPA 스프링 데이터 JPA
이건 그냥 고대의 선배님들이 이렇게 했다..
편안하게 공부하는 마음으로 했다!
이제는 잘 안씀!!!
1. H2 데이터베이스 설치
→개발이나 테스트 용도로 가볍고 편리한 DB, 웹 화면 제공
https://www.h2database.com/html/download-archive.html
1.4.200버전으로 설치하기!!! 안그러면 오류
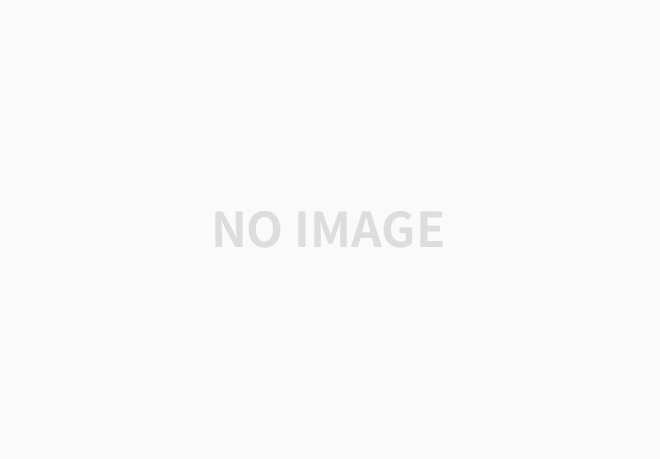
최초에는 데이터베이스 파일 만들어야함
연결누르면
Unsupported database file version or invalid file header in file "
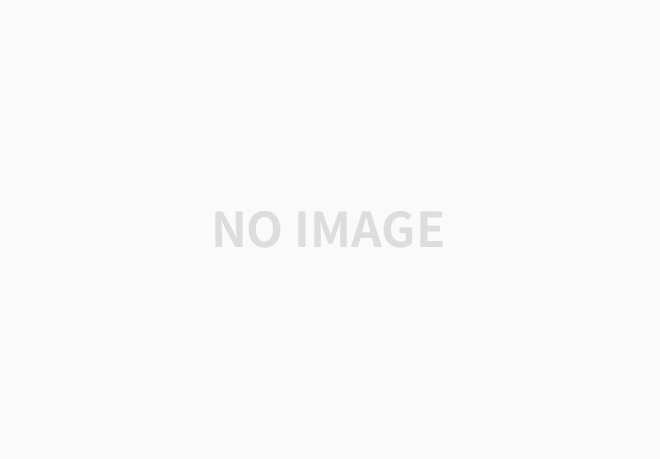
오엥?
지원되지 않는 헤더라길래 test말고 testDB로 치니까 연결됨
데이터베이스 파일 생성 방법
jdbc:h2:~/testDB (최초 한번) ~/test.mv.db 파일 생성 확인 이후부터는 jdbc:h2:tcp://localhost/~/testDB 이렇게 접속
테이블 생성
drop table if exists member CASCADE;
create table member
(
id bigint generated by default as identity,
name varchar(255),
primary key (id)
);
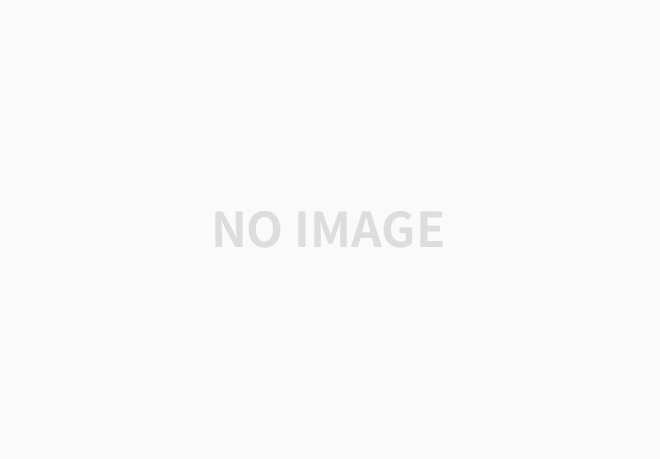
insert into member(name) values ('해연')
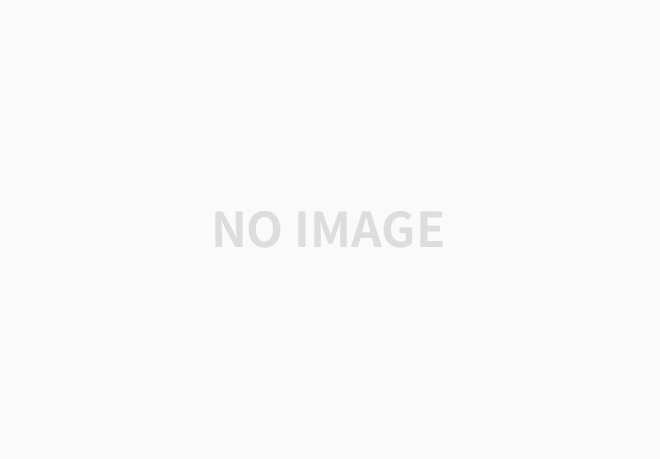
..와 진짜 단순하고 쉽다…………………….
와!!!!신세계네….짱신기해…
꿀팁! sql 파일 따로 관리해주기
꿀팁! sql 파일 따로 관리해주기
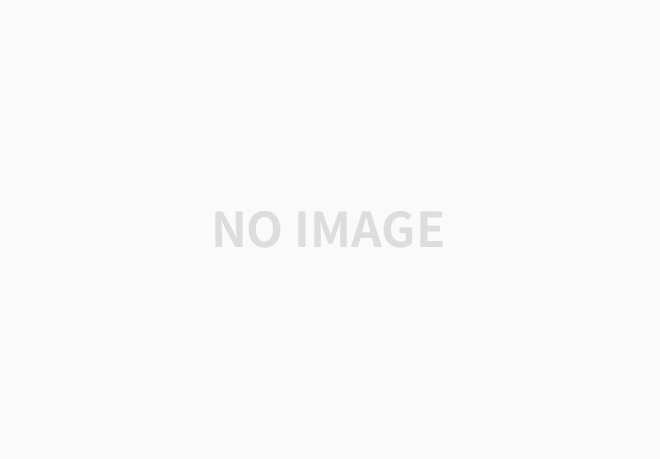
*참고로 이거 끄면 안된다
JDBC 연결
주의! 이렇게 JDBC API로 직접 코딩하는 것은 20년 전 이야기이다. 따라서 고대 개발자들이 이렇게 고생하고 살았구나 생각하고, 정신건강을 위해 참고만 하고 넘어가자.
build.gradle 파일에 jdbc, h2 데이터베이스 관련 라이브러리 추가
implementation 'org.springframework.boot:spring-boot-starter-jdbc'
runtimeOnly 'com.h2database:h2'
스프링 부트 데이터베이스 연결 설정 추가
resources/application.properties
spring.datasource.url=jdbc:h2:tcp://localhost/~/test
spring.datasource.driver-class-name=org.h2.Driver
spring.datasource.username=sa
main/java.repository/JdbcMemberRepository
package hello.hellospring.repository;
import hello.hellospring.domain.Member;
import org.springframework.jdbc.datasource.DataSourceUtils;
import javax.sql.DataSource;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
public class JdbcMemberRepository implements MemberRepository {
private final DataSource dataSource;
public JdbcMemberRepository(DataSource dataSource) {
this.dataSource = dataSource;
}
@Override
public Member save(Member member) {
String sql = "insert into member(name) values(?)"; //파라미터 바인딩 -> 물음표
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = getConnection();
pstmt = conn.prepareStatement(sql,
Statement.RETURN_GENERATED_KEYS); //DB인서트 할 때 값 가져올 때 씀!
pstmt.setString(1, member.getName()); //
pstmt.executeUpdate();
rs = pstmt.getGeneratedKeys();
if (rs.next()) {
member.setId(rs.getLong(1));
} else {
throw new SQLException("id 조회 실패");
}
return member;
} catch (Exception e) {
throw new IllegalStateException(e);
} finally {
close(conn, pstmt, rs);
}
}
@Override
public Optional<Member> findById(Long id) {
String sql = "select * from member where id = ?";
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setLong(1, id);
rs = pstmt.executeQuery();
if(rs.next()) {
Member member = new Member();
member.setId(rs.getLong("id"));
member.setName(rs.getString("name"));
return Optional.of(member);
} else {
return Optional.empty();
}
} catch (Exception e) {
throw new IllegalStateException(e);
} finally {
close(conn, pstmt, rs);
}
}
@Override
public List<Member> findAll() {
String sql = "select * from member";
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = getConnection();
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
List<Member> members = new ArrayList<>();
while(rs.next()) {
Member member = new Member();
member.setId(rs.getLong("id"));
member.setName(rs.getString("name"));
members.add(member);
}
return members;
} catch (Exception e) {
throw new IllegalStateException(e);
} finally {
close(conn, pstmt, rs);
}
}
@Override
public Optional<Member> findByName(String name) {
String sql = "select * from member where name = ?";
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, name);
rs = pstmt.executeQuery();
if(rs.next()) {
Member member = new Member();
member.setId(rs.getLong("id"));
member.setName(rs.getString("name"));
return Optional.of(member);
}
return Optional.empty();
} catch (Exception e) {
throw new IllegalStateException(e);
} finally {
close(conn, pstmt, rs);
}
}
private Connection getConnection() {
return DataSourceUtils.getConnection(dataSource);
}
private void close(Connection conn, PreparedStatement pstmt, ResultSet rs)
{
try {
if (rs != null) {
rs.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (pstmt != null) {
pstmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (conn != null) {
close(conn);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
private void close(Connection conn) throws SQLException {
DataSourceUtils.releaseConnection(conn, dataSource); //이걸로 닫아줘야함
}
}
그냥 아 이렇게도 했었구나~ 정도로만 이해해주자!
service/SpringConfig
private DataSource dataSource;
@Autowired //데이터베이스 연결 할 수 있도록
public SpringConfig(DataSource dataSource) {
this.dataSource = dataSource;
}
에러발생 - 아 DB 끄면 안된다구!
org.h2.jdbc.JdbcSQLNonTransientConnectionException: Connection is broken: "java.net.ConnectException: Connection refused: connect: localhost" [90067-214]
다시 켜주니

DB연결이 잘 되었다 ^_^
개방-폐쇄 원칙(OCP, Open-Closed Principle)
→ 확장에는 열려있고, 수정, 변경에는 닫혀있다.
- 스프링의 DI (Dependencies Injection)을 사용하면 기존 코드를 전혀 손대지 않고, 설정만으로 구현( 아까처럼 알아서 인젝션 주입해줌!)
- 클래스를 변경할 수 있다.
확장
새로운 타입을 추가
새로운 기능을 추가
새로운 타입(클래스, 모듈, 함수)을 추가함으로써 기능을 확장
변경
확장이 발생
상위 레벨이 영향을 미치지 않아야 하는 것
확장이 발생했을 때
변경이 발생하지 않는 것
이 원칙을 지키기위한 구현 방법은 ?
- 확장 될 것과 변경을 엄격히 구분한다.
- 이 두 모듈이 만나는 지점에 인터페이스를 정의한다.
- 구현에 대한 의존보다는 정의된 인터페이스를 의존하도록 코드를 작성한다.
- 변경이 발생하는 부분을 추상화하여 분리한다.